What is Git? Think of Git as a tool that takes snapshots of your work. Every time you make a change to your project, Git can take a picture of it. What is GitHub? You can imagine GitHub as a place on the internet where you can save these snapshots. You can also invite your friends or colleagues so they can also access these snapshots and help you make changes, etc.
Git and GitHub serve important roles in software development, enabling collaboration and version control in a way that’s crucial for both individuals and teams. Here’s why we need Git and GitHub:
Git:
- Version Control: Git is a distributed version control system that allows developers to track changes in their code over time. It provides a history of changes, making it easier to understand the evolution of a project.
- Collaboration: Multiple developers can work on the same project simultaneously. Git allows them to merge their changes, ensuring that the codebase remains consistent.
- Branching: Git allows developers to create branches for different features or bug fixes. This enables them to work on these features independently without affecting the main codebase until they’re ready to merge.
- Local Commits: Developers can make local commits without affecting the main repository. This is valuable for experimenting with new features or making quick fixes.
- Code Review: Git facilitates code review through pull requests, allowing developers to review each other’s code and provide feedback before merging changes into the main codebase.
GitHub:
- Remote Repository: GitHub is a web-based platform that hosts Git repositories in the cloud. This makes it easier for developers to collaborate with others, as they can access the code from anywhere with an internet connection.
- Collaboration: GitHub provides tools for issue tracking, project management, and collaboration among team members. It’s widely used for coordinating tasks and communicating about code changes.
- Pull Requests: Developers can create pull requests on GitHub to propose changes, which are then reviewed by peers. This helps maintain code quality and ensures that changes do not introduce new issues.
- Continuous Integration: GitHub integrates with various continuous integration (CI) tools, automating the testing and deployment of code changes. This ensures that code is continuously tested and verified.
- Community and Open Source: GitHub is a hub for open source projects, making it easier for developers to contribute to and collaborate on open source software. It fosters a sense of community and allows people to share their work with the world.
- Documentation: GitHub provides tools for creating and hosting documentation for projects, making it easier for developers and users to understand and work with the software.
Although the focus here will be Git and GitHub, as with most things, there are alternatives. Instead of Git there is Mercurial, Subversion (SVN), and Bazaar. Or GitLab and Bitbucket instead of GitHub.
By the end of this article you will be able to understand the importance of why we as software developers need to use some form of version control. You will learn the most common Git commands that we use everyday, and you’ll setup your GitHub account. It’s free to do so, and honestly, I see it as a MUST HAVE thing if you want to be a software developer. It’s where you’ll keep all your personal projects that you can use to show potential employers. Let’s get started!
How To Use GitHub
Now, just a heads up. This isn’t going to be an in-depth look into GitHub and all of its features. I’m just going to go over the basics and arm you with enough knowledge and (hopefully) enough curiosity to explore further yourself.
Creating A GitHub Account
Head on over to https://github.com and choose ‘sign up’ in the top right hand corner.

Now that you have your account setup and have logged in, you should see a similar screen to this, but you won’t have the list of repositories (repos for short) on the left.
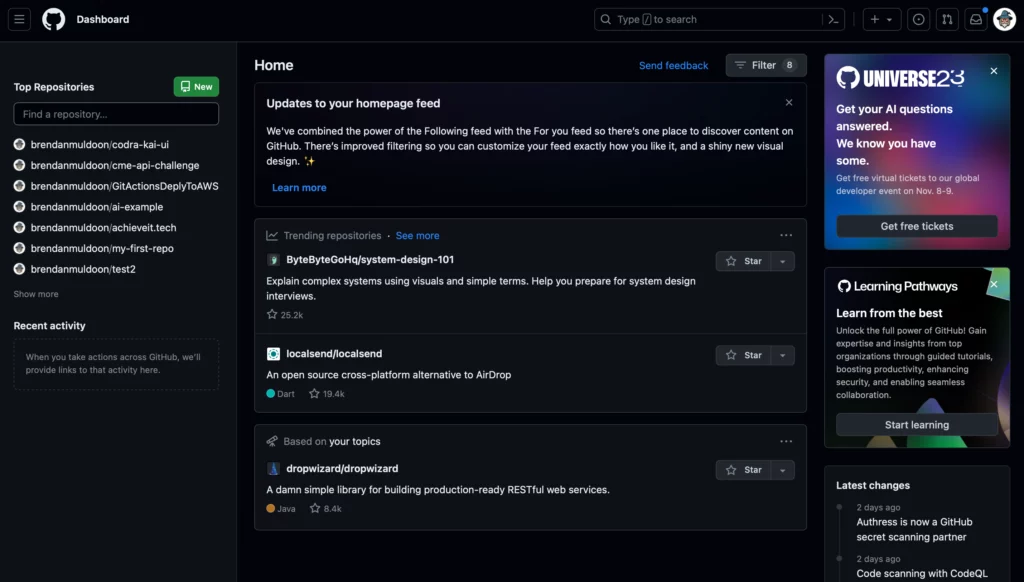
What I want you to do is click ‘new’ on the left to setup a new repo. You will see the below.
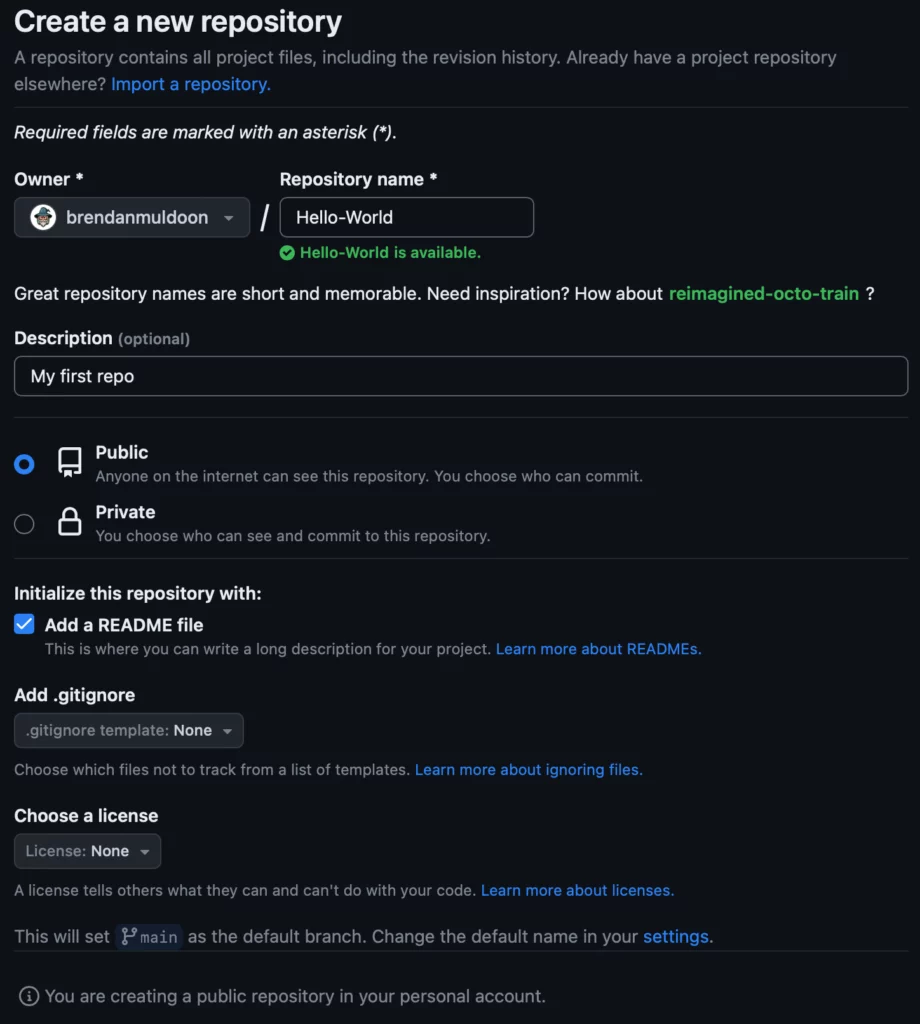
The options available are self-explanatory. Give your repo a name of “Hello-World” and a description of “My first repo”. You can keep it as public or you can set it to private, up to you! Lastly, initialise your repo with a README (this will add a starting file for us). Congratulations on setting up your first repo!
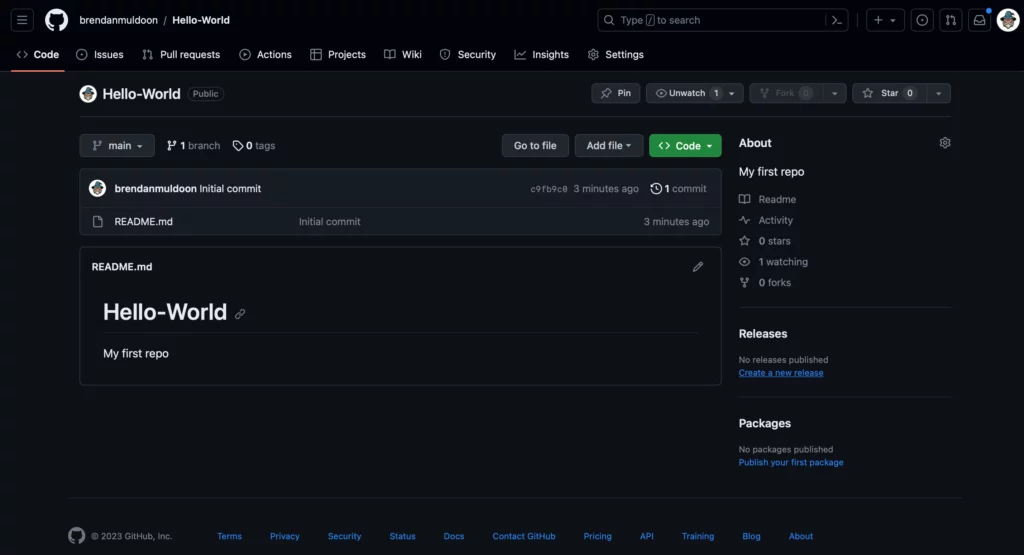
GitHub Branches
By default, you will have a “main” branch created for you. Working directly on the “master” or “main” (same thing, though “main” is now the preferred term) branch in Git is generally discouraged for a few reasons:
- Lack of Isolation: The “main” branch is typically considered the main and stable branch. If you make changes directly to it and those changes have issues or are incomplete, it can disrupt the stability of your project for others.
- Collaboration Issues: If you’re working with others on the same project, making changes directly to “main” can lead to conflicts and make it difficult to coordinate efforts.
- Losing History: It’s harder to keep a clean history of your changes when you make them directly on “main.” Git is designed to allow you to work on new features or bug fixes on separate branches, making it easier to track changes and collaborate with others.
- No Safety Net: Creating branches to work on new features or fixes provides a safety net. If something goes wrong or your changes don’t work as expected, you can abandon the branch without affecting the “main” branch.
To avoid these issues, it’s recommended to create feature branches or bug fix branches to work on your changes and only merge them into “main” when they are complete and tested. This way, you maintain a clean and organised history of your project while also allowing others to collaborate more effectively.
To that end, I want you to create a new branch called “MyFirstBranch”. To do so click on “main”, type in the name of the branch, and click “Create branch MyFirstBranch”. This will create a branch off of the “main” branch. So any changes you make in your new branch are isolated to it and cannot affect anything else.
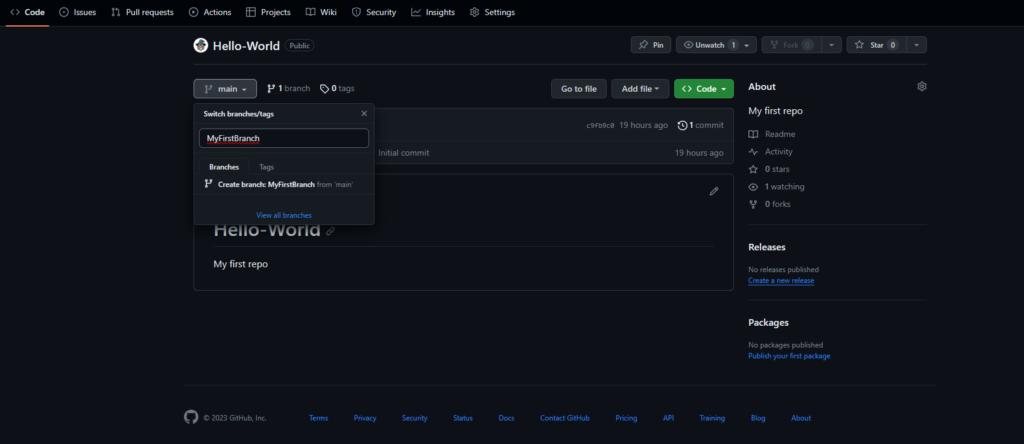
Common GitHub Terminology And What They Mean
Before moving on I just want to pause for a minute. I’ve compiled a list of common terms used in GitHub. Hopefully this will be helpful in providing a little bit more context.
Term | Description |
Repository (Repo) | A repository is a container for your project, where all your project’s files and revision history are stored. Repositories can be public or private. |
Clone | Cloning a repository means creating a local copy of a remote repository on your computer. This local copy allows you to work with the repository’s files. |
Fork | Forking a repository means making a personal copy of someone else’s repository. It’s a common way to contribute to open source projects. You can make changes to your fork and submit pull requests to the original repository. |
Branch | A branch is a separate line of development within a repository. You can create branches to work on specific features or fixes without affecting the main codebase. |
Commit | A commit is a snapshot of the changes you’ve made to your project. Commits create a history of your project’s development. |
Pull Request (PR) | A pull request is a way to propose changes to a repository. It allows you to compare the changes in your branch to the base branch and request that your changes be merged. |
Merge | Merging is the process of integrating changes from one branch into another. Pull requests are often merged to incorporate the proposed changes. |
Issues | Issues are used to track bugs, enhancements, and tasks related to a project. They are often used for project management and collaboration. |
Star | Starring a repository is a way to bookmark it. It helps you keep track of interesting projects and notifies you of updates. |
Watch | Watching a repository means you’ll receive notifications for activity on that repository, including issues, pull requests, and comments. |
Readme | The README is a file (typically README.md) that provides information about the project, including its purpose, how to use it, and other relevant details. |
Collaborators | Collaborators are individuals who have write access to a repository and can push changes. Repository owners can grant this access to others. |
Organization | An organization on GitHub is a way to group repositories and users under a common name, typically used by companies, open source projects, and communities. |
GitHub Actions | GitHub Actions is a feature that allows you to automate your workflows. You can use it for tasks like building, testing, and deploying your code. |
GitHub Pages | GitHub Pages is a feature that allows you to publish web pages directly from your GitHub repository. |
How To Use Git
Disclaimer! You are not going to be an expert in using Git at the end of this guide… sorry. But you will have enough information to use the common everyday commands. In this section you’re going to learn the following.
How to:
- Clone your new repo
- Switch to a different branch and make some changes in it
- Add your changes
- Commit your changes
- Push your changes
Before that though, we need a way to interact with GitHub from our computer. If you’re on Linux or macOS you by default will already have Git. Verify this buy opening the terminal and running the following command:
git --version
However, for Windows users we need to download “Git Bash”. In short, Git Bash is a command-line tool that provides a Linux-like environment on Windows, making it easier for users to work with Git and various command-line utilities.
Downloading Git Bash For Windows
Take yourself on over to: Git – Downloads (git-scm.com) and download the latest version for Windows. Honestly, just follow the installation instructions and you can’t go wrong.

When everything is finished, you should have access to the Git Bash app. It will look like this:
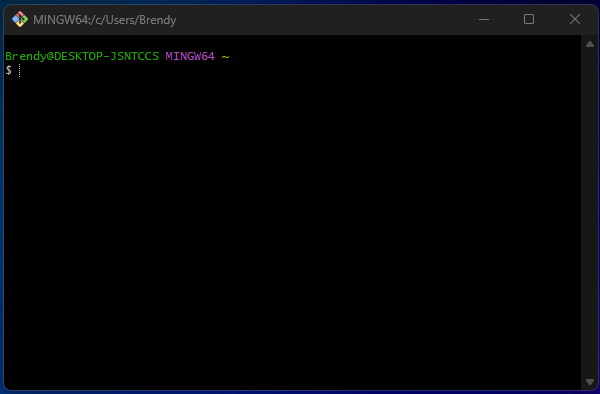
Firstly, you should configure your username and email address. These settings are to identify you as the author of commits. Match these to what you used on GitHub:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Clone
Okay, so lets run our first command and clone our Hello-World repo on GitHub. On the right, there is a green button “Code”, click that and copy the URL that is shown.

Where you save your code is completely up to you. You can do it on your Desktop, in your Documents, it doesn’t matter. But if I was you, I would maybe save it in a dedicated “code” folder for all your projects. This isn’t mandatory, just personal advice.
Open up Git Bash, navigate to the folder where you want to save your code, for me that’s in a folder called “demo code”. Remember, use the command “cd” to change directory. You now want to run the following command:
// template
git clone <your repo url>
// example
git clone https://github.com/brendanmuldoon/Hello-World.git
You should see something similar to the below
$ git clone https://github.com/brendanmuldoon/Hello-World.git
Cloning into 'Hello-World'...
remote: Enumerating objects: 3, done.
remote: Counting objects: 100% (3/3), done.
remote: Total 3 (delta 0), reused 0 (delta 0), pack-reused 0
Receiving objects: 100% (3/3), done.
What has happened here is that git has created a new folder for me inside my “demo code” folder and called it “Hello-World”, the same name as my repo on GitHub. Now, cd into that folder
cd Hello-World
The eagle eyed among you will notice at the end in brackets
(main)
By default we will be placed in the “main” branch, but we want to switch to the new branch we made.
Switch
To switch to a different branch simply run the following
// template
git switch <name of the branch to switch to>
//example
git switch MyFirstBranch
You should see the brackets have now changed to (MyFristBranch) and see the output below:
Switched to a new branch 'MyFirstBranch'
branch 'MyFirstBranch' set up to track 'origin/MyFirstBranch'.
Add
Lets make a change to the README file that was pulled down from our repo. Open it up with any of your favourite text editors. I’m just going to use notepad, but feel free to use anything. Now type the following: “My first commit”. Save it and close.
Something I like to do, to remind me of the current state of my project, and before I start any commits, is running the following command:
git status
This should return this:
On branch MyFirstBranch
Your branch is up to date with 'origin/MyFirstBranch'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: README.md
no changes added to commit (use "git add" and/or "git commit -a")
This is basically telling me that, I have modified a file and I have not “staged” it for “commit”. In Git, a “stage” refers to the process of preparing changes in your working directory to be committed to the version control system (GitHub). This preparation is done using the “git add” command, and the area where these changes are temporarily stored is known as the “staging area” or “index.”
You can now do one of the following:
// add all
git add .
// add specific files
git add <path to file> <path to file> .. etc
//example
$ git add README.md
If you run git status you should see something like this:
On branch MyFirstBranch
Your branch is up to date with 'origin/MyFirstBranch'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: README.md
Commit
Now that our changes have been staged we can commit them. This is where we take a snapshot of the changes that are currently in the staging area. We also add a meaningful message to go along with the commit to remind ourselves of what we done or changed. It also helps our team in quickly understanding what you done without looking at the code.
// template
git commit -m "Add your meaningful message here"
// example
git commit -m "Update readme"
There are a lot of blogs online that talk about what should go into a git commit message, and how it should be structured. So I will leave a link for you to explore a little further: Commit message guidelines (github.com).
Push
Lastly, the final piece to the puzzle, pushing our commit to the repo on GitHub for the world to see (unless you set it to private).
git push
You should be asked to sign in to GitHub. If you’re logged in on your browser then just select “Sign in with your browser”.
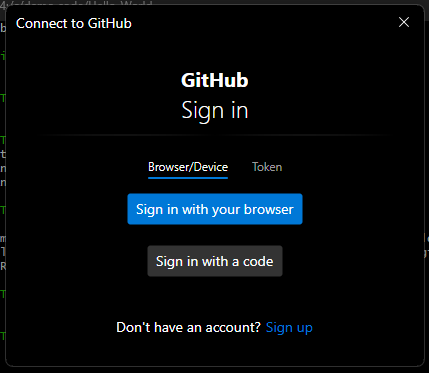
After you have logged in, you should see:
info: please complete authentication in your browser...
Enumerating objects: 5, done.
Counting objects: 100% (5/5), done.
Writing objects: 100% (3/3), 301 bytes | 75.00 KiB/s, done.
Total 3 (delta 0), reused 0 (delta 0), pack-reused 0
To https://github.com/brendanmuldoon/Hello-World.git
c9fb9c0..f63a7c2 MyFirstBranch -> MyFirstBranch
Now if you go back to your branch on GitHub you should see your recent commit:

And that’s it! If you remember these simple commands, you’re already 90% of the way there. Very rarely do I need to use other commands in my day to day life as a developer:
git clone <url>
git status
git add .
git commit -m "Add new cool feature"
git push
Raising A Pull Request (PR)
Once we have finished with all the changes in our branch, we need to raise a request to “merge” the branch into the branch we branched off of. In our case it was the “main” branch. If you were to do this in a development team, you would assign the PR to a senior developer (but doesn’t always have to be). This will be used to conduct a “code review,” in which you will receive feedback and possibly identify areas where you could improve.
However, this is our own personal repo, so we can obviously skip all of that. From the previous picture, I want you to click on “Compare & pull request”. You will be brought to this screen:

Here you can assign it to someone to review and a bunch of other things. But for now we just want to click “Create pull request”.
GitHub will analyse the PR and make sure that there will be no merge conflicts with the base branch (“main”). Merge conflicts occur when GitHub is unable to automatically combine changes from different branches into a single, coherent codebase. Common reasons for merge conflicts are:
- Concurrent Changes to the Same Lines: If two or more people make changes to the same lines of code in different branches, Git won’t know which changes to keep, leading to a conflict.
- Branch Divergence: When a branch has diverged significantly from its base branch (often the “master” or “main” branch), and both branches contain changes that affect the same code, merging can result in conflicts.
- Deletion Conflicts: If one branch deletes a file or a specific block of code, while another branch modifies or attempts to delete the same file or block, Git doesn’t know what to do, leading to a conflict.
- Renaming or Moving Files: When a file is renamed or moved in one branch, and another branch makes changes to the original file, Git can’t automatically determine the relationship between the old and new file names.
- Whitespace and Formatting Conflicts: In some cases, conflicts can occur due to differences in whitespace (e.g., tabs vs. spaces, line endings) or code formatting (indentation, code style).
- Binary Files: Git primarily works with text files. When binary files (e.g., images, compiled executables) are changed in multiple branches, Git can’t resolve the differences, leading to conflicts.
- Unmerged Commits: If you have already merged changes from one branch into another and there are still unmerged commits in the source branch, these unmerged commits can cause conflicts when you attempt to merge again.
- Complex Merge Histories: In projects with complex branching and merging strategies, it can be challenging for Git to track the relationships between branches and their common ancestors. This complexity can lead to conflicts.
- Manual Edits or Forced Resolutions: Sometimes, developers may manually edit or force certain changes during the merge resolution process. If these manual edits conflict with other changes, it can result in additional merge conflicts.
To resolve merge conflicts, you’ll need to open the conflicted files, manually edit them to include the desired changes, and then commit the resolved files. It’s essential to carefully review and test the changes to ensure the merged code functions correctly after the conflict resolution. Additionally, effective communication within your development team can help reduce the frequency of conflicts by coordinating changes and understanding each other’s work.

For us though, we can go ahead and click “Merge pull request” -> “Confirm merge”. You can choose to keep the branch or you can click “Delete branch” to remove it. Sometimes it’s worth keeping around just in case you need to go back and make more changes.
And that’s it! Thank you for sticking aorund to the end. If you found this helpful, then you might find some of my other articles helpful too. Please consider giving them a read!